Player
Players are Entities that represents the individual behind the mouse and keyboard. Players are spawned automatically when connected to the server.
👪Inheritance
This class shares methods and events from Base Entity.
Authority
🟥 You cannot spawn or Destroy Players.
Examples​
-- Spawns and possess a Character when a Player joins the server
Player.Subscribe("Spawn", function(player)
local new_char = Character(Vector(), Rotator(), "helix::SK_Male")
player:Possess(new_char)
end)
-- Destroys the Character when the Player leaves the server
Player.Subscribe("Destroy", function(player)
local character = player:GetControlledCharacter()
if (character) then
character:Destroy()
end
end)
Static Functions​
Inherited Entity Static Functions
Base Entityscripting-reference/classes/base-classes/Entity
Returns | Name | Description | |
---|---|---|---|
![]() | table of Base Entity | GetAll | Returns a table containing all Entities of the class this is called on |
![]() | Base Entity | GetByIndex | Returns a specific Entity of this class at an index |
![]() | integer | GetCount | Returns how many Entities of this class exist |
![]() | iterator | GetPairs | Returns an iterator with all Entities of this class to be used with pairs() |
![]() | table | Inherit | Inherits this class with the Inheriting System |
![]() | table of table | GetInheritedClasses | Gets a list of all directly inherited classes from this Class created with the Inheriting System |
![]() | table or nil | GetParentClass | Gets the parent class if this Class was created with the Inheriting System |
![]() | boolean | IsChildOf | Gets if this Class is child of another class if this Class was created with the Inheriting System |
![]() | function | Subscribe | Subscribes to an Event for all entities of this Class |
![]() | function | SubscribeRemote | Subscribes to a custom event called from server |
![]() | Unsubscribe | Unsubscribes all callbacks from this Event in this Class within this Package, or only the callback passed |
Functions​
Inherited Entity Functions
Base Entityscripting-reference/classes/base-classes/Entity
Returns | Name | Description | |
---|---|---|---|
![]() | integer | GetID | Gets the universal network ID of this Entity (same on both client and server) |
![]() | table | GetClass | Gets the class of this entity |
![]() | boolean | IsA | Recursively checks if this entity is inherited from a Class |
![]() | function | Subscribe | Subscribes to an Event on this specific entity |
![]() | function | SubscribeRemote | Subscribes to a custom event called from server on this specific entity |
![]() | Unsubscribe | Unsubscribes all callbacks from this Event in this Entity within this Package, or only the callback passed | |
![]() | SetValue | Sets a Value in this Entity | |
![]() | any | GetValue | Gets a Value stored on this Entity at the given key |
Destroy | Destroys this Entity | ||
![]() | boolean | IsValid | Returns true if this Entity is valid (i.e. wasn't destroyed and points to a valid Entity) |
CallRemoteEvent | Calls a custom remote event directly on this entity to a specific Player | ||
CallRemoteEvent | Calls a custom remote event directly on this entity | ||
BroadcastRemoteEvent | Calls a custom remote event directly on this entity to all Players |
Returns | Name | Description | |
---|---|---|---|
Ban | Bans the player from the server | ||
Connect | Redirects the player to another server | ||
Kick | Kicks the player from the server | ||
Possess | Makes a Player to possess and control a Character | ||
![]() | StartCameraFade | Does a camera fade to/from a solid color | |
![]() | SetManualCameraFade | Turns on camera fading at the given opacity | |
![]() | StopCameraFade | Stops camera fading. | |
![]() | SetCameraLocation | Sets the Player's Camera Location (only works if not possessing any Character) | |
![]() | SetCameraRotation | Sets the Player's Camera Rotation | |
![]() | TranslateCameraTo | Smoothly moves the Player's Camera Location (only works if not possessing any Character) | |
![]() | RotateCameraTo | Smoothly moves the Player's Camera Rotation | |
![]() | SetCameraSocketOffset | Sets the Player's Camera Socket Offset (Spring Arm Offset) | |
![]() | SetCameraArmLength | Sets the Player's Camera Arm Length (Spring Arm length) | |
![]() | AttachCameraTo | Attaches the Player`s Camera to an Actor | |
![]() | ResetCamera | Resets the Camera to default state (Unspectate and Detaches) | |
![]() | Spectate | Spectates other Player | |
SetName | Sets the player's name | ||
SetVOIPChannel | Sets the VOIP Channel of this Player (will only communicate with other players in the same channel) | ||
![]() | SetVOIPSetting | Sets the VOIP Settings of this Player | |
![]() | SetVOIPVolume | Sets the VOIP Volume of this Player | |
UnPossess | Release the Player from the Character | ||
![]() | string | GetAccountID | |
![]() | string | GetAccountName | |
![]() | string | GetAccountIconURL | Return a URL which can be used through WebUI and Widgets to display the Player's Steam Avatar (64x64) |
Vector | GetCameraLocation | ||
float | GetCameraArmLength | ||
Rotator | GetCameraRotation | ||
![]() | Character or nil | GetControlledCharacter | |
string | GetIP | ||
![]() | integer | GetDimension | Gets this Player's dimension |
![]() | string | GetName | |
![]() | integer | GetPing | |
![]() | integer | GetVOIPChannel | |
![]() | any | GetValue | |
boolean | IsHost | ||
boolean | IsLocalPlayer | ||
![]() | VOIPSetting | GetVOIPSetting |

Ban
​
Bans the player from the server
my_player:Ban(reason)
Type | Parameter | Default | Description |
---|---|---|---|
string | reason |

Connect
​
Redirects the player to another server
my_player:Connect(IP, password?)

Kick
​
Kicks the player from the server
my_player:Kick(reason)
Type | Parameter | Default | Description |
---|---|---|---|
string | reason |

Possess
​
Makes a Player to possess and control a Character
my_player:Possess(new_character, blend_time?, exp?)
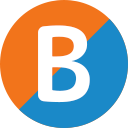
StartCameraFade
​
Does a camera fade to/from a solid color. Animates automatically
my_player:StartCameraFade(from_alpha, to_alpha, duration, to_color, should_fade_audio, hold_when_finished)
Type | Parameter | Default | Description |
---|---|---|---|
float | from_alpha | Alpha at which to begin the fade. Range [0..1], where 0 is fully transparent and 1 is fully opaque solid color. | |
float | to_alpha | Alpha at which to finish the fade. | |
float | duration | How long the fade should take, in seconds. | |
Color | to_color | Color to fade to/from. | |
boolean | should_fade_audio | True to fade audio volume along with the alpha of the solid color. | |
boolean | hold_when_finished | True for fade to hold at the ToAlpha until explicitly stopped (e.g. with StopCameraFade) |
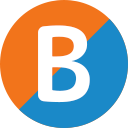
SetManualCameraFade
​
Turns on camera fading at the given opacity. Does not auto-animate, allowing user to animate themselves. Call StopCameraFade to turn fading back off.
my_player:SetManualCameraFade(in_fade_amount, color, in_fade_audio)
Type | Parameter | Default | Description |
---|---|---|---|
float | in_fade_amount | Range [0..1], where 0 is fully transparent and 1 is fully opaque solid color. | |
Color | color | ||
boolean | in_fade_audio |
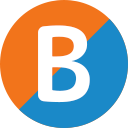
StopCameraFade
​
Stops camera fading.
my_player:StopCameraFade()
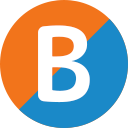
SetCameraLocation
​
Sets the Player's Camera Location (only works if not possessing any Character)
my_player:SetCameraLocation(location)
Type | Parameter | Default | Description |
---|---|---|---|
Vector | location |
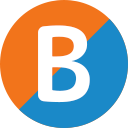
SetCameraRotation
​
Sets the Player's Camera Rotation
my_player:SetCameraRotation(rotation)
Type | Parameter | Default | Description |
---|---|---|---|
Rotator | rotation |
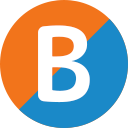
TranslateCameraTo
​
Smoothly moves the Player's Camera Location (only works if not possessing any Character)
my_player:TranslateCameraTo(location, time, exp?)
Type | Parameter | Default | Description |
---|---|---|---|
Vector | location | ||
float | time | ||
float | exp? | 0 | Exponential used to smooth interp, use 0 for linear movement |
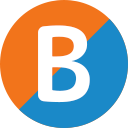
RotateCameraTo
​
Smoothly moves the Player's Camera Rotation
my_player:RotateCameraTo(rotation, time, exp?)
Type | Parameter | Default | Description |
---|---|---|---|
Rotator | rotation | ||
float | time | ||
float | exp? | 0 | Exponential used to smooth interp, use 0 for linear movement |
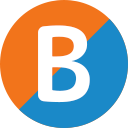
SetCameraSocketOffset
​
Sets the Player's Camera Socket Offset (Spring Arm Offset)
my_player:SetCameraSocketOffset(socket_offset)
Type | Parameter | Default | Description |
---|---|---|---|
Vector | socket_offset |
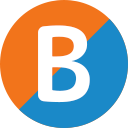
SetCameraArmLength
​
Sets the Player's Camera Arm Length (Spring Arm length)
my_player:SetCameraArmLength(length, force)
Type | Parameter | Default | Description |
---|---|---|---|
float | length | ||
boolean | force | Whether to bypass interpolation and set the target to its value directly |
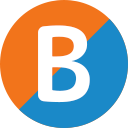
AttachCameraTo
​
Attaches the Player`s Camera to an Actor
my_player:AttachCameraTo(actor, socket_offset, blend_speed)
Type | Parameter | Default | Description |
---|---|---|---|
Base Actor | actor | ||
Vector | socket_offset | ||
float | blend_speed |
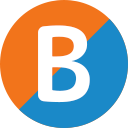
ResetCamera
​
Resets the Camera to default state (Unspectate and Detaches)
my_player:ResetCamera()
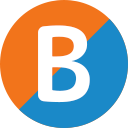
Spectate
​
Spectates other Player
my_player:Spectate(player, blend_speed?)

SetName
​
Sets the player's name
my_player:SetName(new_name)
Type | Parameter | Default | Description |
---|---|---|---|
string | new_name |

SetVOIPChannel
​
Sets the VOIP Channel of this Player (will only communicate with other players in the same channel)
my_player:SetVOIPChannel(channel)
Type | Parameter | Default | Description |
---|---|---|---|
integer | channel |
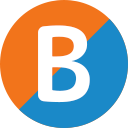
SetVOIPSetting
​
Sets the VOIP Settings of this Player
my_player:SetVOIPSetting(setting)
Type | Parameter | Default | Description |
---|---|---|---|
VOIPSetting | setting |
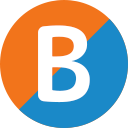
SetVOIPVolume
​
Sets the VOIP Volume of this Player
my_player:SetVOIPVolume(volume)
Type | Parameter | Default | Description |
---|---|---|---|
float | volume |

UnPossess
​
Release the Player from the Character
my_player:UnPossess()
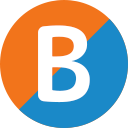
GetAccountID
​
— Returns string.
local ret = my_player:GetAccountID()
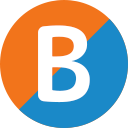
GetAccountName
​
— Returns string.
local ret = my_player:GetAccountName()
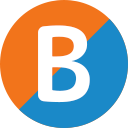
GetAccountIconURL
​
Return a URL which can be used through WebUI and Widgets to display the Player's Steam Avatar (64x64)
— Returns string.
local ret = my_player:GetAccountIconURL()

GetCameraLocation
​
— Returns Vector.
local ret = my_player:GetCameraLocation()

GetCameraArmLength
​
— Returns float.
local ret = my_player:GetCameraArmLength(return_base?)
Type | Parameter | Default | Description |
---|---|---|---|
boolean | return_base? | false | Whether to return the current (false) or base (true) value. The base is the same value set by SetCameraArmLength(). |

GetCameraRotation
​
— Returns Rotator.
local ret = my_player:GetCameraRotation()
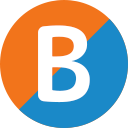
GetControlledCharacter
​
local ret = my_player:GetControlledCharacter()

GetIP
​
— Returns string.
local ret = my_player:GetIP()
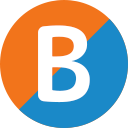
GetDimension
​
Gets this Player's dimension
— Returns integer.
local ret = my_player:GetDimension()
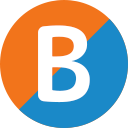
GetName
​
— Returns string.
local ret = my_player:GetName()
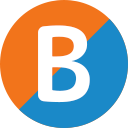
GetPing
​
— Returns integer.
local ret = my_player:GetPing()
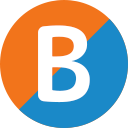
GetVOIPChannel
​
— Returns integer.
local ret = my_player:GetVOIPChannel()
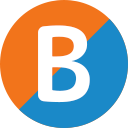
GetValue
​
— Returns any.
local ret = my_player:GetValue(key, fallback)

IsHost
​
— Returns boolean.
local ret = my_player:IsHost()

IsLocalPlayer
​
— Returns boolean.
local ret = my_player:IsLocalPlayer()
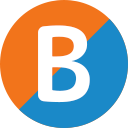
GetVOIPSetting
​
— Returns VOIPSetting.
local ret = my_player:GetVOIPSetting()
Events​
Inherited Entity Events
Base Entityscripting-reference/classes/base-classes/Entity
Name | Description | |
---|---|---|
![]() | Spawn | Triggered when an Entity is spawned/created |
![]() | Destroy | Triggered when an Entity is destroyed |
![]() | ValueChange | Triggered when an Entity has a value changed with :SetValue() |
![]() | ClassRegister | Triggered when a new Class is registered with the Inheriting System |
Name | Description | |
---|---|---|
DimensionChange | Triggered when a Player changes it's dimension | |
![]() | Possess | Trigerred when Player starts controlling a Character |
Ready | Triggered when Player is ready (the client fully joined, loaded the map and all entities and is ready to play) | |
![]() | UnPossess | A Character was released from the Player |
![]() | VOIP | When a Player starts/ends using VOIP |

DimensionChange
​
Triggered when a Player changes it's dimension
Player.Subscribe("DimensionChange", function(self, old_dimension, new_dimension)
-- DimensionChange was called
end)
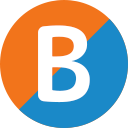
Possess
​
Trigerred when Player starts controlling a Character
Player.Subscribe("Possess", function(self, character)
-- Possess was called
end)

Ready
​
Triggered when Player is ready (the client fully joined, loaded the map and all entities and is ready to play)
Player.Subscribe("Ready", function(self)
-- Ready was called
end)
Type | Argument | Description |
---|---|---|
Player | self |
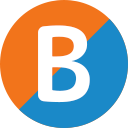
UnPossess
​
A Character was released from the Player
Player.Subscribe("UnPossess", function(self, character)
-- UnPossess was called
end)
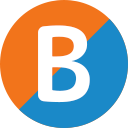
VOIP
​
When a Player starts/ends using VOIP
When 'is_talking' is true, return false to prevent this voice stream from playing (on server will prevent for everyone, on client will prevent only for the client)
Player.Subscribe("VOIP", function(self, is_talking)
-- VOIP was called
end)